Shell Navigation in .Net Maui
Hi guys, In this blog, we will learn the basics of Shell Navigation in .Net Maui.
Shell navigation includes URI-based navigation which uses routes to navigate from one page to another.
If you want to learn Navigation through NavigationPage click here for my blog on Navigation through NavigationPage.
Table of Content
- Prerequisite
- Simple Navigation
- Passing data with Navigation
- Navigating Back or Removing Page
- Clearing Navigation Stack or Changing MainPage
Prerequisite
Before we make sure you have add all your pages in AppShell.xaml
Add your pages as ShellContent inside the Shell tag as shown below.
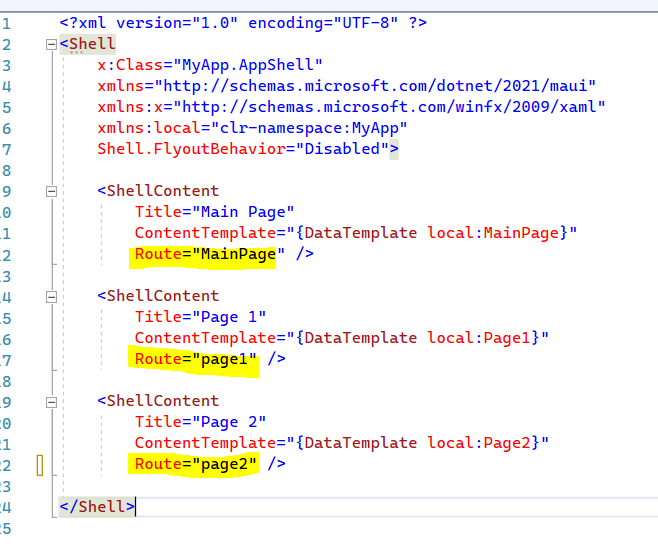
An important thing to notice here is the Route, this is the route we will use in the future for shell navigation.
Open App.cs and set MainPage as AppShell

Now Open MauiProgram.cs & register your routes for shell navigation as shown below.

Simple Navigation
Here we will navigate from one page to another, The basic simple navigation.
For that, we will use the Shell class’s Current object's GoToAsync method.
Page1 → Page2
await Shell.Current.GoToAsync(“page2”);
GoToAsync method takes the route for the navigation which we Registered in MauiProgram.cs
Passing data with navigation
To pass data from Page1 to Page2 we can simply use query parameters while navigating as shown below.

string data = “Data from main page.”;
await Shell.Current.GoToAsync($”page2?data={data}”);
Processing data passed to Page2
In the Page2 where data is passed using QueryProperty
QueryProperty(“Your_Binding_Property_Name” , ”Query_Parameter_Name”)
To Send multiple data in shell navigation use the below syntax
string data = “Data from main page.”;
bool hasAdditionalData = true;
await Shell.Current.GoToAsync($”page2?data={data}&hasMoreData={hasAdditionalData}”);
I would recommend sending Dictionary as data, as it’s easy to pass complex objects.
Navigating Back
To navigate back in shell navigation simply use “..” in GoToAsync.
Page2 ← Page1
await Shell.Current.GoToAsync(“..”);
To pass data while navigating back we can use the same query parameter in URI.
await Shell.Current.GoToAsync($“..?data={data}”);
We can also remove the current page from the navigation stack while navigating from one page to another.
await Shell.Current.GoToAsync(“../page3”);
Before “../page3” our navigation stack will look like the below.
MainPage → Page1 → Page2
After “../page3” our navigation stack will look like the below.
MainPage → Page1 → Page3
Clearing the navigation stack or changing MainPage
This is similar to PopToRootAsync or Setting a new MainPage.
await Shell.Current.GoToAsync(“//page1”);
OR
await Shell.Current.GoToAsync(“///page1”);
This will clear the navigation stack & sets Page1 as a MainPage in the navigation.
Happy Coding :D